Get Current Location In Angular
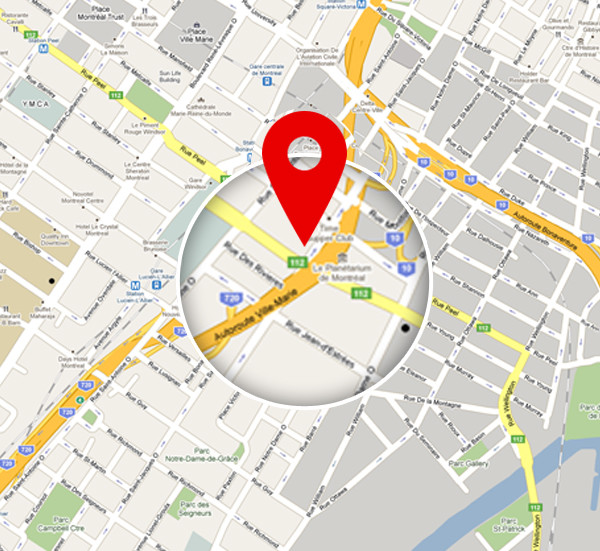
Introduction:
In this article, we are going to learn how to add Google maps in an Angular project. The steps are as follows. below steps :
Step 1:
Using the below command, create the Angular App.
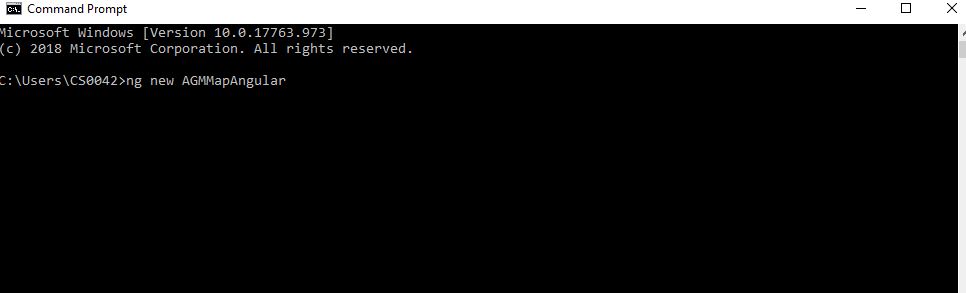
Step 2:
Open the project which you have created in Visual Studio code and install the agm library using the following command “npm i @agm/core”.
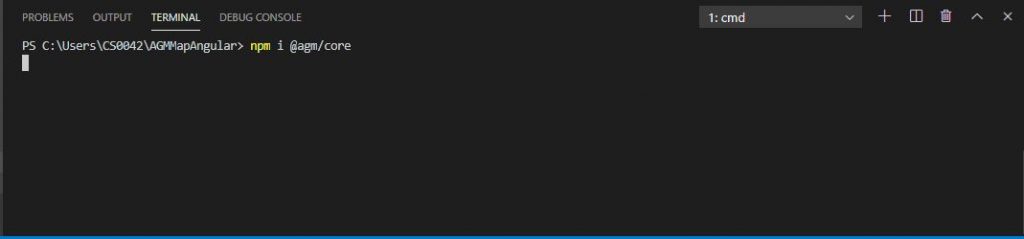
Step 3:
Install the Google map reference npm using the following command.
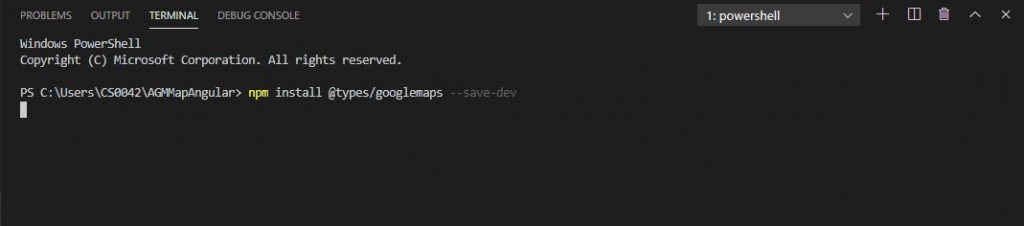
Step 4:
Open the tsconfig.app.json in your project and update the following reference code.
{
"extends": "./tsconfig.json",
"compilerOptions": {
"outDir": "./out-tsc/app",
"types": ["googlemaps"]
},
Whats is tsconfig.app.json?
It holds the root files and the compiler options.
Step 5:
Like the above steps, open tsconfig.spec.json file in your project and update the following reference code.
{
"extends": "./tsconfig.json",
"compilerOptions": {
"outDir": "./out-tsc/spec",
"types": [
"jasmine",
"node",
"googlemaps"
]
},
What is tsconfig.spec.json?
File has a setting to convert your typescript code into javascript code to make the browser understand our code.
Step 6:
Now open your app.module.ts file & add the agm library file using the following line:
import { AgmCoreModule } from '@agm/core';
How To Get Map Api Key:
A) Visit Google developer console page or click here
B) Open the menu & click the APIs & Services and select “Credentials”

C) On the credentials page, open the “CREATE CREDENTIALS” and select the API key.
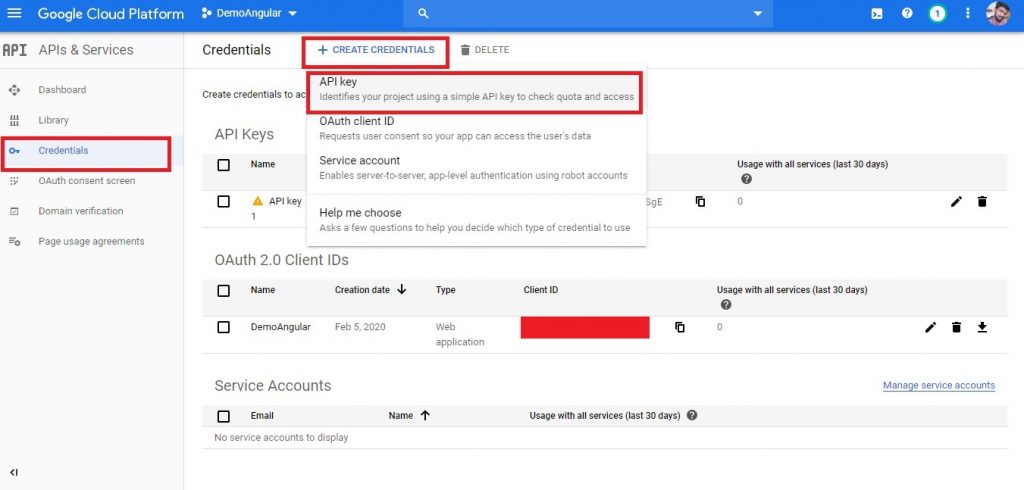
D) Once the process is done, the API code will be generated and can be seen in the list of new APIs in the same credentials page.
Step 7:
imports: [
FormsModule,
BrowserModule,
AppRoutingModule,
AgmCoreModule.forRoot({
apiKey: 'Paste Your Google Key'
}),
],

Step 8:
Open the app.component.ts file which you can add in the agmmap reference file.
import {AgmMap, MouseEvent,MapsAPILoader } from '@agm/core';
A) After then we can declare the agmmap for view child.
@ViewChild(AgmMap,{static: true}) public agmMap: AgmMap;
B) And assign the mapsAPIloader in constructor.
constructor( private apiloader:MapsAPILoader)
{
}
Step 10:
Now, open app.component.html file & add the below code. Similarly, open the app.component.ts file.
<form>
<agm-map style="height: 300px;" [latitude]="lat" [longitude]="lng" [zoom]="zoom" (mapClick)="mapClicked($event)">
<agm-marker [latitude]="lat" [longitude]="lng"></agm-marker>
</agm-map>
</form>
Let us open the app.component.ts file by adding the following code which is used to get the current latitude and longitude.
After getting latitude and longitude, we need to convert it into a real time address.
get(){
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition((position: Position) => {
if (position) {
this.lat = position.coords.latitude;
this.lng = position.coords.longitude;
this.getAddress=(this.lat,this.lng)
console.log(position)
this.apiloader.load().then(() => {
let geocoder = new google.maps.Geocoder;
let latlng = {lat: this.lat, lng: this.lng};
geocoder.geocode({'location': latlng}, function(results) {
if (results[0]) {
this.currentLocation= results[0].formatted_address;
console.log(this.assgin);
} else {
console.log('Not found');
}
});
});
}
})
}
}
In order to check the latitude and longitude, we need to call the method (Step 10) to ngOnInit to capture the address of that particular latitude and longitude.
ngOnInit()
{
this.get()
this.agmMap.triggerResize(true);
this.zoom = 16;
}
Whats is ngOnInit?
It’s called once the component is initialized.
Whats is Zoom?
Zoom customizes visibility.
Step 13:
Now, add the click event in map and get the latitude and longitude address. Then convert the formatted address using below code .
mapClicked($event: MouseEvent) {
this.latitude= $event.coords.lat,
this.longitude= $event.coords.lng
this.apiloader.load().then(() => {
let geocoder = new google.maps.Geocoder;
let latlng = {lat: this.latitude, lng: this.longitude};
geocoder.geocode({'location': latlng}, function(results) {
if (results[0]) {
this.currentLocation = results[0].formatted_address;
console.log(this.currentLocation);
} else {
console.log('Not found');
}
});
});
}
Step 14:
After completing all the above steps, we can run the project using the below command.
Command – ng serve –open
Step 15:
Now, the project will run successfully. In order to open the Project in local browser, we need to provide the location permission in the browser.
Summary:
I hope you understand the way, How to add Google Map in Angular Project along with current location and map click event. Any feedback or query related to this article is most welcome and this project source code available visit to git or click here .