Passing Value One Activity To Another Activity
Let’s start,
Step 1 : Open Visual Studio->New Project->Templates->Visual C#-> Android->Blank App, then select Blank App. Give Project Name and Project Location.
Step 2 : Next we need to create Second page, so go to Solution Explorer->Project Name->Resources->layout. After that right click on Add->New Item, then open new Dialog box.
Step 3 : Select Android Layout and give name Secondpage.axml.
Step 4 : And then we need to add one more Activity, so again go to Solution Explorer, then Project Name. After that right click to Add->New Item, then open new Dialog box.
Step 5 : Then select Activity and give name it SecondActivity.cs.
Step 6 : Next go for Solution Explorer-> Project Name->Resources->layout->Main.axml click to open Design View.
Step 7 : Select Toolbar Drag and Drop Button, TextView and PlainText.
XML Code
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent"> <TextView android:text="FIRST PAGE" android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/textView1" /> <EditText android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/txtpassvalue" /> <Button android:text="Send" android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/btnsend" /> </LinearLayout>
Step 8 : Next open Solution Explorer-> Project Name->Resources->layout->Secondpage.axml click to open Design View.
Step 9 : Select Toolbar Drag and Drop, Button and TextView.
XML Code
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <Button android:text="GET VALUE" android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/btngetvalue" /> <TextView android:textAppearance="?android:attr/textAppearanceLarge" android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/txtgetvalue" /> </LinearLayout>
Step 10 : Next open Solution Explorer-> Project Name->MainActivity.cs click to open Code Window, then give following code,
C# Code
using System; using Android.App; using Android.Content; using Android.Runtime; using Android.Views; using Android.Widget; using Android.OS; namespace DataValuePass { [Activity(Label = "DataValuePass", MainLauncher = true, Icon = "@drawable/icon")] public class MainActivity: Activity { EditText txtdatapass; Button btnsend; protected override void OnCreate(Bundle bundle) { base.OnCreate(bundle); SetContentView(Resource.Layout.Main); btnsend = FindViewById < Button > (Resource.Id.btnsend); txtdatapass = FindViewById < EditText > (Resource.Id.txtpassvalue); btnsend.Click += Button_Click; } private void Button_Click(object sender, EventArgs e) { var intent = new Intent(this, typeof(SecondActivity)); intent.PutExtra("DATA_PASS", txtdatapass.Text); //DATA_PASS is Identify the Value Pass variable this.StartActivity(intent); } } }
Step 11 : Next to open Solution Explorer-> Project Name->SecondActivity.cs click to open Code Window, then give following code,
C# Code
using System; using System.Collections.Generic; using System.Linq; using System.Text; using Android.App; using Android.Content; using Android.OS; using Android.Runtime; using Android.Views; using Android.Widget; namespace DataValuePass { [Activity(Label = "SecondActivity")] public class SecondActivity: Activity { Button btngetvalue; TextView txtgevalue; string txtvalues; protected override void OnCreate(Bundle savedInstanceState) { base.OnCreate(savedInstanceState); SetContentView(Resource.Layout.Secondpage); txtvalues = Intent.GetStringExtra("DATA_PASS"); //Get The Value btngetvalue = FindViewById < Button > (Resource.Id.btngetvalue); txtgevalue = FindViewById < TextView > (Resource.Id.txtgetvalue); btngetvalue.Click += Btngetvalue_Click;; } private void Btngetvalue_Click(object sender, EventArgs e) { txtgevalue.Text = txtvalues; } } }
Step 12 : Press F5 or Build and Run the Application,
- First Screen Give the Text Box Value “Hi Welcome” then Click Send Button.Next Move Second Screen.
- Second Screen to Press Get Button to Retrieve the passing value of “Hi Welcome” to display TextView
- .
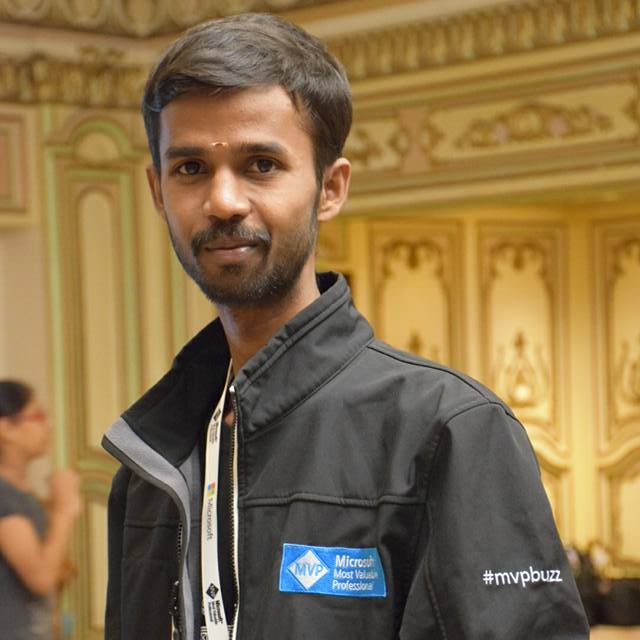
Anbu Mani(Microsoft MVP) is working Software Engineer in Changepond Technologies, Chennai, Tamilnadu, India. Having 4+ years of experience and his area of interest is C#, ASP.NET, SQL Server, Xamarin and Xamarin Forms,Azure…etc