Excel File Export In Angular Using Service
What is Angular?
- Angular is a Client side JavaScript Framework which allows us to create Reactive Client-side Application.
- Angular provides an approach to create a Single Page Application. By default, it supports Two-way Data binding.
Why should we have uses of Services in Angular?
- Angular service methods can be invoked from any component of Angular, like Controllers, Directives etc.
- This helps in dividing the web application into small, different logical units which can be reused.
We use the @Injectable class decorators to automatically resolve and inject all the other classes. This only works if each parameter has a TypeScript type associated with it, which the DI framework uses as the token.
What is a dependency?
- When module A in an application needs module B to run, then module B is dependent to module A i.e. module B is a dependency of module A.
- Dependency Injection is a way of architecting an application which makes the code to re-use, easier to test and easier to maintain.
- Angular comes with a Dependency Injection (DI) framework of its own and it can be used wherever it is required.
Implement Dependency Injection in Angular:
Below are the ways to implement Dependency Injection in Angular.
- Using @Injectable() in the service
- Using @NgModules() in the module
- Using Providers in the component
Step 1 – Create an Angular Project:
Create an Angular Project,
The command to create a new Angular project “ng new ”. Let’s create an Angular project using the following commands.
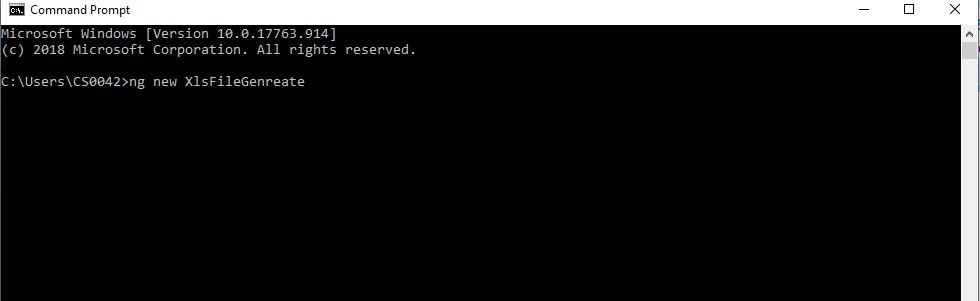
Step 2 – Create a Service:
Open the above created project in Visual Studio code and Create service by using the following command “ng generate service <myService>”

Step 3
Create a JSON file from any location Ex [D:/yourfilename.json]. Store the array values once JSON is created.
{
"Cardetails": [
{
"Id": 1,
"CarName": "Hummer",
"Model": "H3",
"Mileage": "196321",
"Color": "Pink"
},
{
"Id": 2,
"CarName": "Chevrolet",
"Model": "1500 Silverado",
"Mileage": "195310",
"Color": "Blue"
},
{
"Id": 3,
"CarName": "Infiniti",
"Model": "M",
"Mileage": "194846",
"Color": "Puce"
},
}
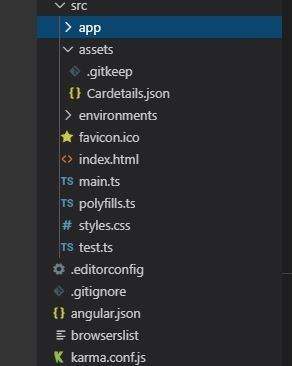
Step 4
Open the created service and add angular/http service by using the following command npm install @angular/http, using he following code.
import {Http} from '@angular/http';
export class {
constructor(
private http:Http
)
{ }
Step 5
Get the stored JSON file through the service that you have created.
Getdata() {
return this.http.get("assets/Cardetails.json").map(res => {
return res.json()
});
}
}
Step 6
Add the below code in the file app.module.ts.
import {
HttpModule
} from '@angular/http'
import {
XlsServiceService
}
rom './xls-service.service'
imports: [
HttpModule
],
providers: [
XlsServiceService
],
Step 7
Add the below code snippet to the file “app.component.ts”
import{
XlsServiceService
} from './xls-service.service'
ngOnInit()
{
this.Getdetails()
}
Getdetails(){
this.excelservice.Getdata().subscribe(check=>
{
this.listdetails=check.Cardetails
});
}
Step 8
Install file-saver and xlsx through the commands “npm install file-saver” and “npm install xlsx”. Now, open the “excel.service.ts” and paste the following code.
import * as FileSaver from 'file-saver';
import * as XLSX from 'xlsx';
Step 9
Declare file type and file extension with below code.
const EXCEL_TYPE = 'application/vnd.openxmlformats-officedocument.spreadsheetml.sheet;charset=UTF-8';
const EXCEL_EXTENSION = '.xlsx';
Step 10
Code to export JSON in Excel File
public exportAsExcelFile(json: any[], excelFileName: string): void {
const worksheet: XLSX.WorkSheet = XLSX.utils.json_to_sheet(json);
console.log('worksheet', worksheet);
const workbook: XLSX.WorkBook = {
Sheets: {
'data': worksheet
},
SheetNames: ['data']
};
const excelBuffer: any = XLSX.write(workbook, {
bookType: 'xlsx',
type: 'array'
});
this.saveAsExcelFile(excelBuffer, excelFileName);
}
private saveAsExcelFile(buffer: any, fileName: string): void {
const data: Blob = new Blob([buffer], {
type: EXCEL_TYPE
});
FileSaver.saveAs(data, fileName + '_export_' + new Date().getHours() + ':' + new Date().getMinutes() + ':' + new Date().getSeconds() + EXCEL_EXTENSION);
}
Step 11
Open the file “app.component.html” and paste the following code to see the HTML template
<button(click)="Exportelx()">GenreateXls</button>
Step 12
In HTML page, add a button to call a click function to export an excel file which calls the method in service.
Exportelx() {
this.excelservice.exportAsExcelFile(this.listdetails, "Carinformation")
}
Step 13
To see the output in browser, use the following command “ng serve –open”
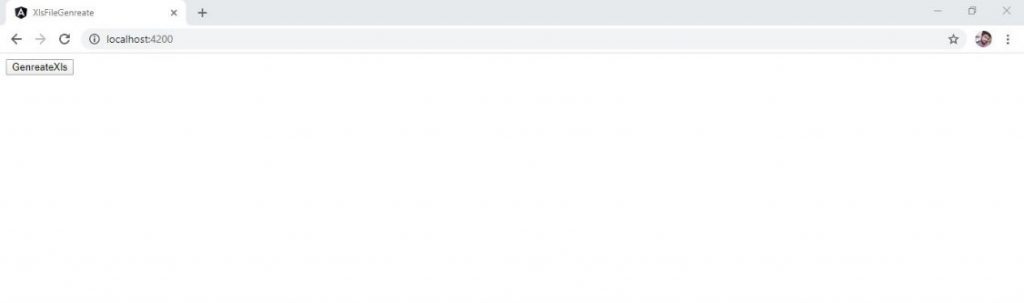
Summary
We learned how to export Excel files in Angular applications.

Hello this is Haridhass, working as Software Developer in Congenial Studioss for more than 2+ years. Skilled in Angular,C#.NET, NodeJs,SQL Server,WebApi,Html,CSS,JavaScript,TypeScript