Angular Decimal Validation
Introduction Angular Directives
Directives are instructions to the DOM.
There are three types of directive
- Component
- Structural
- Attribute
Component:
Directives with a template.
Structural :
Change the DOM layout by adding and removing DOM elements.(leading with *).
Attribute :
Change the appearance or behavior of an element.
List of Angular Directives:
- *ngIf The NgIf directive is used when you want to display or remove an element based on a condition. If the condition is false the element the directive is attached to will be removed from
- Syntax : *ngIf=”<condition>”
- *ngFor Its point is to repeat a given HTML template once for each value in an array, each time passing it the array value as context for string interpolation or binding.
- Syntax: <li *ngFor=”let user of listuser”>
- ngClass The NgClass directive allows you to set the CSS class dynamically for a DOM element.
- ngStyle The NgStyle directive lets you set a given DOM elements style properties.
- ngSwitch This directive allows us to render different elements depending on a given condition.
Step 1:
Let’s create an Angular project using following npm command
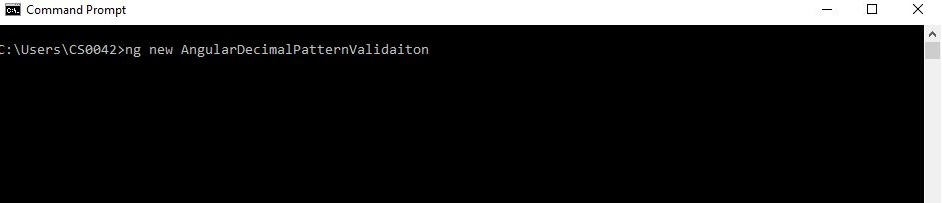
Step 2:
Open the created project in Visual Studio code and generate directive by using below command

Step 3:
open the created decimalvalidation.directive.ts
Selector:
- Selector tells Angular to create and insert an instance of this component where it finds <product-desc> tag. For example, if an app’s HTML contains <product-desc></product-desc>, then Angular inserts an instance of the Product Description view between those tags
- Your HTML just uses it as Attribute for an element
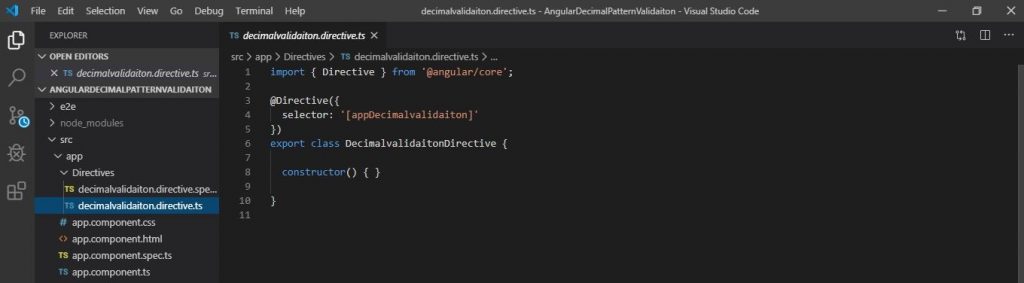
Step 4:
Let’s open the decimalvalidation.directive.ts Use the below regular expression code for Decimal Validation,
import { Directive,ElementRef,HostListener } from '@angular/core';
@Directive({
selector: '[appDecimalvalidaiton]'
})
export class DecimalvalidaitonDirective {
//Declare regularExpression
private regex: RegExp = new RegExp(/^\d*\.?\d{0,2}$/g);
//Declare key
private specialKeys: Array<string> = ['Backspace', 'Tab', 'End', 'Home', '-', 'ArrowLeft', 'ArrowRight', 'Del', 'Delete'];
constructor(
private el: ElementRef
)
{ }
@HostListener('keydown', ['$event'])
//
onKeyDown(event: KeyboardEvent) {
console.log(this.el.nativeElement.value);
// Allow Backspace, tab, end, and home keys
if (this.specialKeys.indexOf(event.key) !== -1) {
return;
}
//Check the datetype
let current: string = this.el.nativeElement.value;
const position = this.el.nativeElement.selectionStart;
const next: string = [current.slice(0, position), event.key == 'Decimal' ? '.' : event.key, current.slice(position)].join('');
if (next && !String(next).match(this.regex)) {
event.preventDefault();
}
}
}
Regular Expression:
A regular expression is an object that describes a pattern of characters. Regular expressions are used to perform pattern-matching and “search-and-replace” functions on text.
ElementRef:
It is a service, which gives us access to the DOM Object. The ElementRef gives the directive direct access to the DOM element upon which it’s attached.
HostListener:
This decorator allows us to subscribe to the events which is raised from the DOM element. Listen to the event occurs on the element and act accordingly. This is a function decorator that accepts an event name. When that event gets fired on the host element it calls the associated function.
keydown:
The keydown event is fired when a key is pressed. Unlike the keypress event, the keydown event is fired for all keys, regardless of whether they produce a character value.
Step 5:
And finally call the directive selector name in Dom page.
<input type="text" maxlength="15" autocomplete="off" appDecimalvalidaiton >
Summary:
In this article, we discussed how to validate decimal pattern using directive